December 14, 2021
Minimal WebAPI in .NET 6
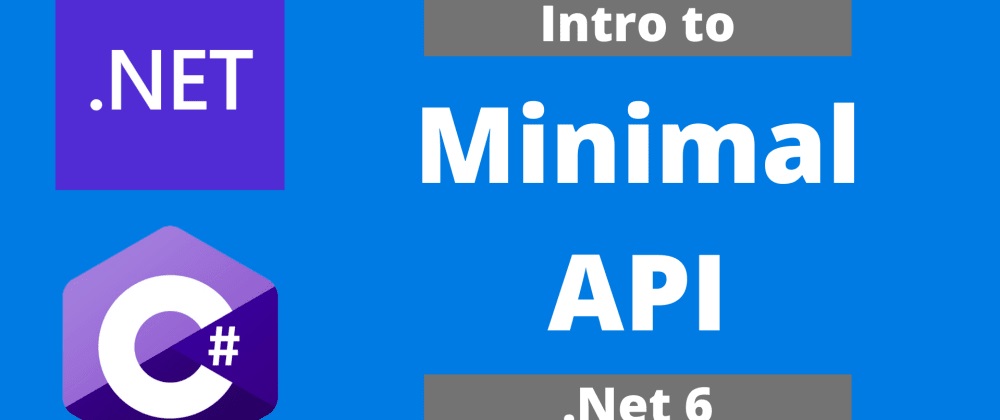
Walk around with minimal WebAPI in .NET 6, a new feature of .NET 6 that provides a new way to build HTTP APIs with minimal dependencies.
Benefit of Minimal API
- Less files: Startup.cs isn’t there anymore, only Program.cs remains.
- Top level statements and implicit global using.
- Its powered by .NET 6 and C# 10 so all of the latest improvements and functionalities are supported out of the box.
- API handled without controllers.
- Best Performance which since the application is so simple a lot of bootstrapping that is required to build and compile the application is simplified which means the application runs much faster.
Let's start
Prerequisites
- Latest .NET 6 SDK (SDK 6.0 and above only).
- Visual Studio 2022 with the ASP.NET and web development workload.
Create the WebAPI
Run this command:
1
dotnet new webapi -minimal -o LinhWork
After it's done, you will see the started code in Program.cs
Program.cs1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
var builder = WebApplication.CreateBuilder(args); // Add services to the container. // Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle builder.Services.AddEndpointsApiExplorer(); builder.Services.AddSwaggerGen(); var app = builder.Build(); // Configure the HTTP request pipeline. if (app.Environment.IsDevelopment()) { app.UseSwagger(); app.UseSwaggerUI(); } app.UseHttpsRedirection(); app.MapGet("/weatherforecast", () => { var forecast = Enumerable.Range(1, 5).Select(index => new WeatherForecast ( DateTime.Now.AddDays(index), Random.Shared.Next(-20, 55), summaries[Random.Shared.Next(summaries.Length)] )) .ToArray(); return forecast; }) .WithName("GetWeatherForecast"); app.Run(); var summaries = new[] { "Freezing", "Bracing", "Chilly", "Cool", "Mild", "Warm", "Balmy", "Hot", "Sweltering", "Scorching" }; record WeatherForecast(DateTime Date, int TemperatureC, string? Summary) { public int TemperatureF => 32 + (int)(TemperatureC / 0.5556); }
They had a big difference with previous version, as you can see, now these are ConfigureServices() and Configure() and Main() method are gone, instead we only had few lines of code without any method in Program.cs.
Let's start to see the detail.
1 2 3 4 5
var builder = WebApplication.CreateBuilder(args); // Add services to the container. // Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle builder.Services.AddEndpointsApiExplorer(); builder.Services.AddSwaggerGen();
It like the ConfigureServices() method before which create the web builder and register services to container.
1 2 3 4 5 6 7 8 9 10
var app = builder.Build(); // Configure the HTTP request pipeline. if (app.Environment.IsDevelopment()) { app.UseSwagger(); app.UseSwaggerUI(); } app.UseHttpsRedirection();
It's exactly what Configure() did before, which configure the HTTP request pipeline for our application.
So how about the controller? In this started project template we can't see the Controller folder and controller class file as well.
Yeah, now controller will be configure like this one
1 2 3 4 5 6 7 8 9 10 11 12 13
app.MapGet("/weatherforecast", () => { var forecast = Enumerable.Range(1, 5).Select(index => new WeatherForecast ( DateTime.Now.AddDays(index), Random.Shared.Next(-20, 55), summaries[Random.Shared.Next(summaries.Length)] )) .ToArray(); return forecast; }) .WithName("GetWeatherForecast");
With the new minimal Web API in .NET 6, we will use the Map{Verb} and MapMethods to configured WebApplication. We can follow this post to know more detail.
1 2 3 4 5 6 7
app.MapGet("/", () => "This is a GET"); app.MapPost("/", () => "This is a POST"); app.MapPut("/", () => "This is a PUT"); app.MapDelete("/", () => "This is a DELETE"); app.MapMethods("/options-or-head", new[] { "OPTIONS", "HEAD" }, () => "This is an options or head request ");
Finally, we can make the simple Hello World with minimal Web API just by a few lines of code.
1 2 3 4 5 6
var builder = WebApplication.CreateBuilder(args); var app = builder.Build(); app.MapGet("/", () => "Hello World"); app.Run();
That's it for now. Keep coding and enjoy exploring !!!
I’m glad you’re here. I like the simple so I created this minimalist site to share what I’ve learned interesting during my journey in technologies and in life. Keep coding and enjoy exploring !!!